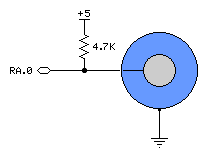 |
|
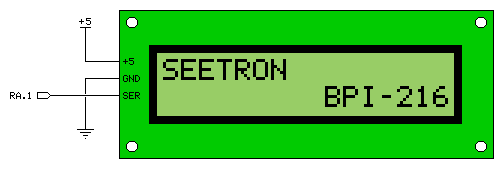 |
' -------------------------------------------------------------------------
' Program Description
' -------------------------------------------------------------------------
'
' This program scans the 1-Wire bus and when a device is detected it will
' read and display its serial number.
' -------------------------------------------------------------------------
' Device Settings
' -------------------------------------------------------------------------
DEVICE SX28, OSCXT2, TURBO, STACKX, OPTIONX
FREQ 4_000_000
ID "1-WIRE"
' -------------------------------------------------------------------------
' IO Pins
' -------------------------------------------------------------------------
DQ VAR RA.0 ' 1-Wire bus (4.7k pull-up)
SOut VAR RA.1 ' output to SEETRON 2x16
' -------------------------------------------------------------------------
' Constants
' -------------------------------------------------------------------------
Baud CON "N9600"
LcdI CON $FE ' instruction
LcdCls CON $01 ' clear the LCD
LcdHome CON $02 ' move cursor home
LcdCrsrL CON $10 ' move cursor left
LcdCrsrR CON $14 ' move cursor right
LcdDispL CON $18 ' shift chars left
LcdDispR CON $1C ' shift chars right
LcdDDRam CON $80 ' Display Data RAM control
LcdCGRam CON $40 ' Character Generator RAM
LcdLine1 CON $80 ' DDRAM address of line 1
LcdLine2 CON $C0 ' DDRAM address of line 2
SearchROM CON $F0
ReadROM CON $33 ' read ID, serial num, CRC
' -------------------------------------------------------------------------
' Variables
' -------------------------------------------------------------------------
idx VAR Byte ' loop counter
char VAR Byte ' char for LCD
owByte VAR Byte ' byte for OW work
owSerial VAR Byte(8) ' OW serial number
tmpB1 VAR Byte ' subroutine work vars
tmpB2 VAR Byte
tmpB3 VAR Byte
tmpW1 VAR Word
' =========================================================================
PROGRAM Start
' =========================================================================
' -------------------------------------------------------------------------
' Subroutine Declarations
' -------------------------------------------------------------------------
DELAY SUB 1, 2 ' delay in milliseconds
TX_BYTE SUB 1, 2 ' transmit byte
TX_STR SUB 2 ' transmit string
TX_HEX2 SUB 1 ' transmit byte as HEX2
' -------------------------------------------------------------------------
' Program Code
' -------------------------------------------------------------------------
Start:
PLP_A = %0011 ' enable pull-ups
PLP_B = %00000000 ' .. except RA.0 and RA.1
PLP_C = %00000000
DELAY 750 ' let LCD initialize
Main:
TX_BYTE LcdI ' clear screen, home cursor
TX_BYTE LcdCls
DELAY 1
TX_STR "SX/B 1-Wire Demo" ' write banner in LCD
Scan_DQ:
TX_BYTE LcdI
TX_BYTE LcdLine2
OWRESET DQ, owByte ' reset and sample presence
ON owByte GOTO Bus_Short, Bad_Bus, Found, No_Device
GOTO Main
Bus_Short:
TX_STR "1-Wire bus short"
DELAY 1000
GOTO Scan_DQ
Bad_Bus:
TX_STR "Bad connection "
DELAY 1000
GOTO Scan_DQ
Found:
TX_STR "Found device "
DELAY 500
TX_BYTE LcdI ' move back to L2/C0
TX_BYTE LcdLine2
OWRESET DQ, owByte ' reset device
OWWRBYTE DQ, ReadROM ' send read ROM command
FOR idx = 0 TO 7 ' read serial number
OWRDBYTE DQ, owByte
owSerial(idx) = owByte
NEXT
FOR idx = 7 TO 0 STEP -1 ' display on LCD
owByte = owSerial(idx)
TX_HEX2 owByte ' -- hex mode
NEXT
DELAY 2000
GOTO Scan_DQ
No_Device:
TX_STR "No device "
GOTO Scan_DQ
' -------------------------------------------------------------------------
' Subroutines
' -------------------------------------------------------------------------
' Use: DELAY ms
' -- 'ms' is delay in milliseconds, 1 - 65535
DELAY:
IF __PARAMCNT = 1 THEN
tmpW1 = __PARAM1 ' save byte value
ELSE
tmpW1 = __WPARAM12 ' save word value
ENDIF
PAUSE tmpW1
RETURN
' -------------------------------------------------------------------------
' Use: TX_BYTE theByte {, count}
' -- transmit "theByte" at "Baud" on "SOut"
' -- optional "count" may be specified (must be > 0)
TX_BYTE:
tmpB1 = __PARAM1 ' save byte
IF __PARAMCNT = 1 THEN ' if no count
tmpB2 = 1 ' set to 1
ELSE ' otherwise
tmpB2 = __PARAM2 ' get count
IF tmpB2 = 0 THEN ' do not allow 0
tmpB2 = 1
ENDIF
ENDIF
DO WHILE tmpB2 > 0 ' loop through count
SEROUT SOut, Baud, tmpB1 ' send the byte
DEC tmpB2 ' decrement count
LOOP
RETURN
' -------------------------------------------------------------------------
' Use: TX_STR [string | label]
' -- "string" is an embedded string constant
' -- "label" is DATA statement label for stored z-String
TX_STR:
tmpW1 = __WPARAM12 ' get offset/base
DO
READ tmpW1, char ' read a character
IF char = 0 THEN EXIT ' if 0, string complete
TX_BYTE char ' send character
INC tmpW1 ' point to next character
LOOP
RETURN
' -------------------------------------------------------------------------
' Use: TX_HEX2 theByte
' -- transmit 'theByte' in HEX2 format
TX_HEX2:
tmpB3 = __PARAM1 ' save byte
char = tmpB3 & %11110000 ' mask high nib
SWAP char ' swap nibs
READ HexDigits + char, char ' high nib to hex char
TX_BYTE char ' send it
char = tmpB3 & %00001111 ' get low nib
READ HexDigits + char, char ' low nib to hex char
TX_BYTE char ' send it
RETURN
' =========================================================================
' User Data
' =========================================================================
HexDigits:
DATA "0123456789ABCDEF"